else if Condition in JavaScript
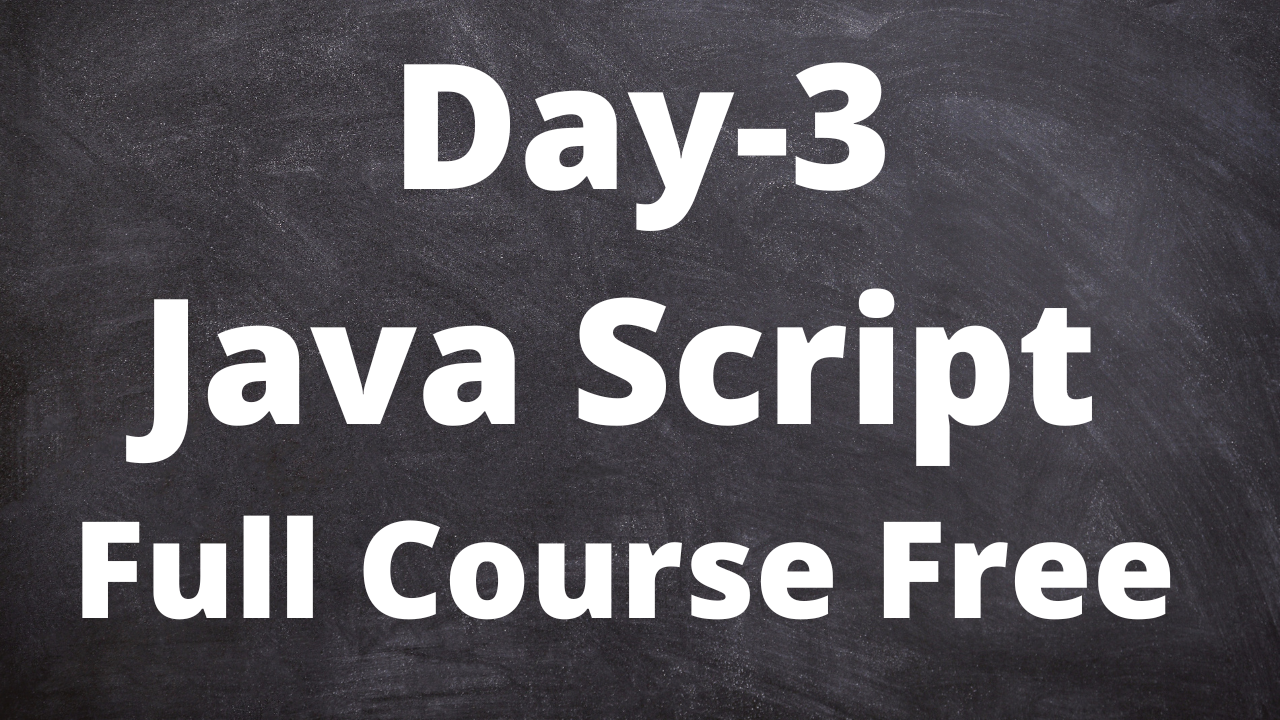
else if Condition in JavaScript
else if statement is useful because it lets us specify a new condition if the first condition is false.
Var Course = 3;
if(Course==1){
document.write(“JAVASCRIPT TUTORIAL”);
} else if(Course==2){
document.write(“HTML TUTORIAL”);
} else{
document.write(“NODE JS”);
}
Output: NODE JS
Note: Final else statement “ends” the else if statement and should be always written after the if and else if statements
Switch: if we need to write multiple conditions then the best practice is we have to choose Switch Case
Like as
Var n = 1
Switch(expression){
Case n1;
statement
break;
The Switch expression is evaluated once. The Value of the expression is compared with the values of each, and if there’s a match, that block of code is executed.
Example: var color = “Yellow”;
Switch (Color){
case”pink”;
document.write(“This is pink”);
break;
case”Grey”;
document.write(“This is Grey”);
break;
case”Black”;
document.write(“This is Black”);
break;
default;
document.write(“color not found”);
Output: color not found
Loop in JavaScript:
In Javascript, there are three types of loops for, while, and do while
For Loop [for( statement1; statement2; statement; )]
Execution Steps
Statement1: statement1 is executed before the loop(the code block) starts
Statement2: define the condition for running the loop(the code block)
Statement3: statement3 is executed each time after the loop (the code block) has been executed.
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all