JavaScript Arithmetic Operators:
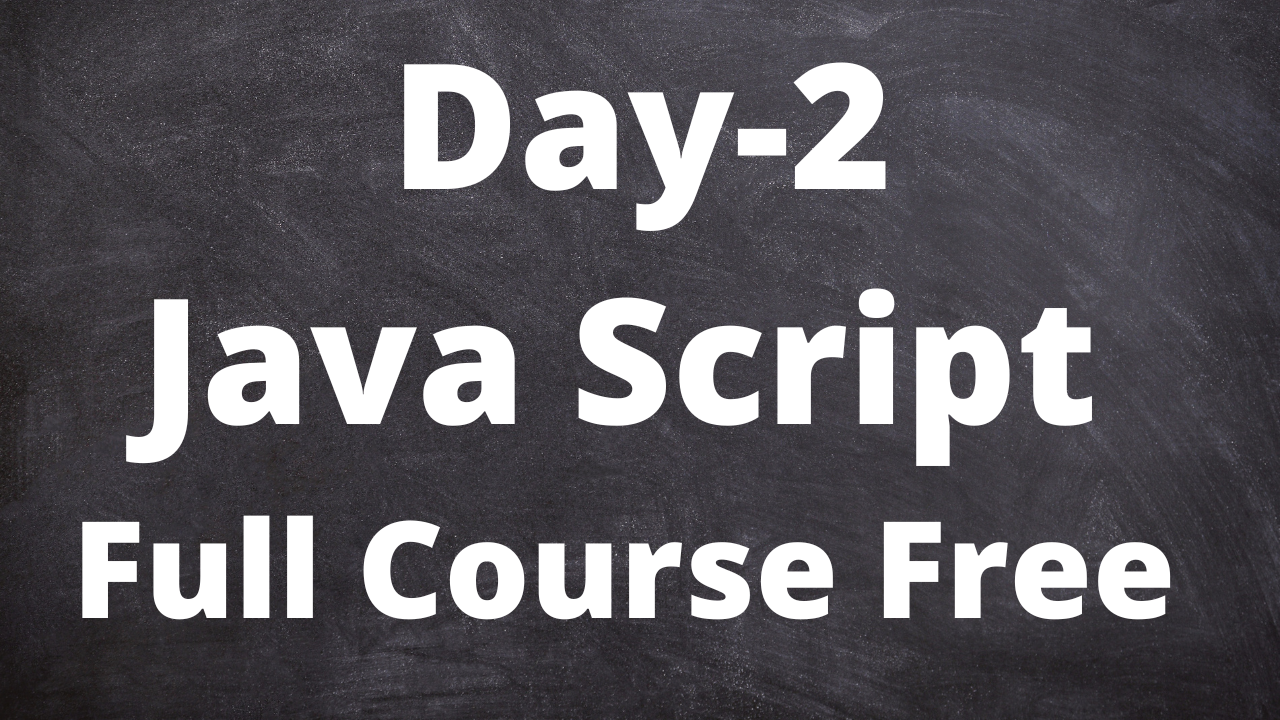
JavaScript Arithmetic Operators:
Operator | Description | Example |
+ | Addition | 10+5=15 |
– | Subtraction | 10-5=5 |
* | Multiplication | 10*5=50 |
/ | Division | 10/5=2 |
% | Moduls | 10%3 = 1 |
++ | Incerement | var billo = 10; billo++ now billo =11 |
— | Decrement | var billo = 10; billo– now billo =9 |
Example1: var b = 20+5;
document.write(b);
output=25
Example2: var b = 20;
var g = b+5+10+80+42+1080
document.write(g);
Note: You can get the result of a string expression using the eval() function, which takes a string expression argument like eval (“50+92*9”) and get output. if the argument will be empty, the output will be undefined.
Operator | Description | Example | Result |
Var++ | Post Incrment | var b=0,g=10; var b= g++ | b=10 and g=11 |
++Var | Pre Increment | var b=0,g=10; var b= ++g | b=11 and g=11 |
Var– | Post Decrement | var b=0,g=10; var b= g– | b=10 and g=9 |
–Var | Pre Decrement | var b=0,g=10; var b= –g | b=9 and g=9 |
JavaScript Assignment Operators:
Operator | Example | is equivalent to |
= | b=g | b=g |
+= | b+=g | b=b+g |
-= | b-=g | b=b-g |
*= | b*=g | b=b*g |
/= | b/=g | b=b/g |
%= | b%=g | b=b%g |
Note: We can use multiple Assignment Operators in one line example: b-=g+=11
JavaScript Comparison Operators:
Var num= 10;
Console.log(num==8);
output: False
Operator | Description | Example | Result |
== | equal to | 5==10 | False |
=== | identical equal to | 5===10 | False |
!= | not equal to | 5!=10 | True |
!== | not identical | 10!==10 | False |
< | less than | 10<5 | false |
<= | less than equal to | 10<=5 | false |
> | greater than | 10>5 | True |
>= | greater than equal to | 10>=5 | True |
JavaScript Logical Operators:
Logical operations, also known as Boolean operators, AND, OR, NOT
Example: Var age=55;
Var isAdult = (age<18)? “Too young”:”Old enough”;
document.write(isAdult);
output: Old enough
JavaScript String Operators:
Var St1 = “I’m Learner”;
Var St1 = “JavaScript with Boomitechie”;
Var St3 = “My Billo”;
document.write(My St1+My St2+My St3);
Note: Numbers in quotes are treated as Strings: For example: “200” is not a number 200 is a String
JavaScript Conditionals and Loops:
if Condition:
if(Condition){
—————
Statements —————
}
Note: Statement will only be executed if the specified condition is true
Var myNum1 = 8;
Var myNum2 = 11;
if(myNum1<myNum2){
alert(“Boomitechie is good website to learn JavaScript”);
}
Else Condition:
We can use the else condition to specify a block of code that will execute if the condition is false
Example: if(Expression){
————–
}
else {
—————
}
if and else statements working together
Var Num1 = 8;
Var Num1 = 11;
if(Num1>Num2){
alert(“this is 1st condition”);
} else{
alert(“this is 2nd condition”);
}
Note: we can go this way also [with the help of operators b>g? alert (b) : alert (g) ]
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all