JavaScript Objects
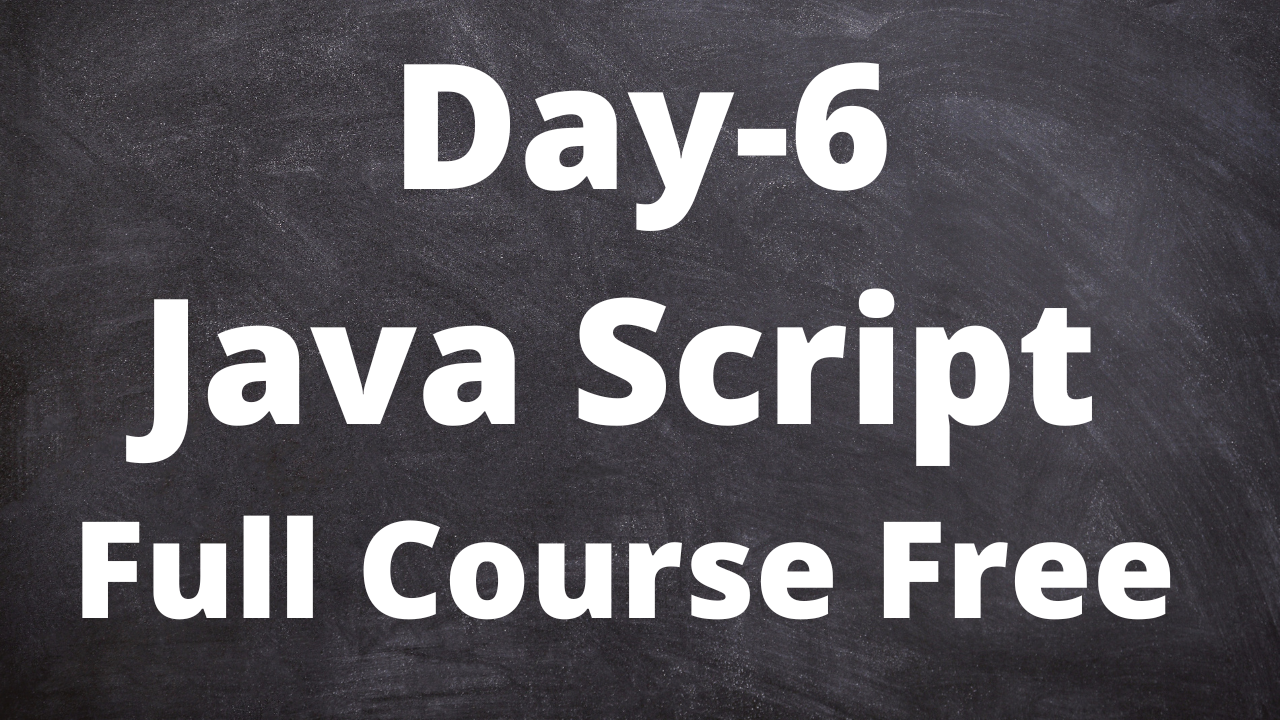
JavaScript Objects
Java Script Variables are containers for data values. Objects are variables too, but they can contain any values. think of an object as a list of values that are written as name: value pairs, with the names and the values separated by colons.
var person = {
name: “Billo”,
favcolor: “green”,
height:160,
age:35
};
Note: JavaScript objects are containers for named values. this example demonstrates how to access the age of our person object
Var person = {
name:”Gunnu”,
favcolor: “green”,
height:172,
age:30
};
var b = person.age
var g = person[“age”];
Output:3030
JavaScript built-in length property is used to can not the number of characters in a property or string
var course = {name: “Js”, lessons: 41};
document.write (course.name.length);
Output:
Note: Object are one of the core concept in JavaScript
Object Methods: An object method is a property that contains a function definition.
Use the following syntax to access an object method.
objectName.methodName()
As you already know, document.write() outputs data.
The write() function is actually a method of the document object.
Note: Methods are functions that are stored as an object property
Object Constructor:
function person(name,age,color){
this.name = name;
this.age =age;
this.favcolor = color;
}
This keyword refers to the current object.
Note: this is not verable. it is a keyword, and its value cannot be chased
Creating Object:
Once you have an object constructor, you can use the new keyword to create new objects of the same type.
Var b = new person(“billo”,35,”green”);
Var g = new person (“gunnu”,30, “Black”);
document.write(b.age);
document.write(g.name);
Output: 35 gunnu
Note: b,g are now object of person type. their properties are assigned to the corresponding values.
Creating Objects:
function person(name,age){
this.name = name;
this.age = age;
}
var Billo = new person(“billo”,35);
var Gunnu = new person(“gunnu”,30);
document.write(Billo.age);
Output: 35
Access the properties of the object by using the dot syntax as we did before.
Object Name | Property’s Name |
Billo | name,age |
Gunnu | name, age |
Object Initialization:
Use the object literal or initializer syntax to create single objects.
var Billo = {name: “billo”,age:35};
var Gunnu = {name: “gunnu”,age:30};
Note: object properties can either contain primitive data types or another object
Object Initialization:
var Billo = {
name: “billo”;
age: 35
};
var Gunnu = {
name: “gunnu”;
age= 30
};
document.write(Billo.age);
Output: 35
Note: don’t forget about the second accessing syntax Billo[‘age’]
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all