JavaScript Arrays:
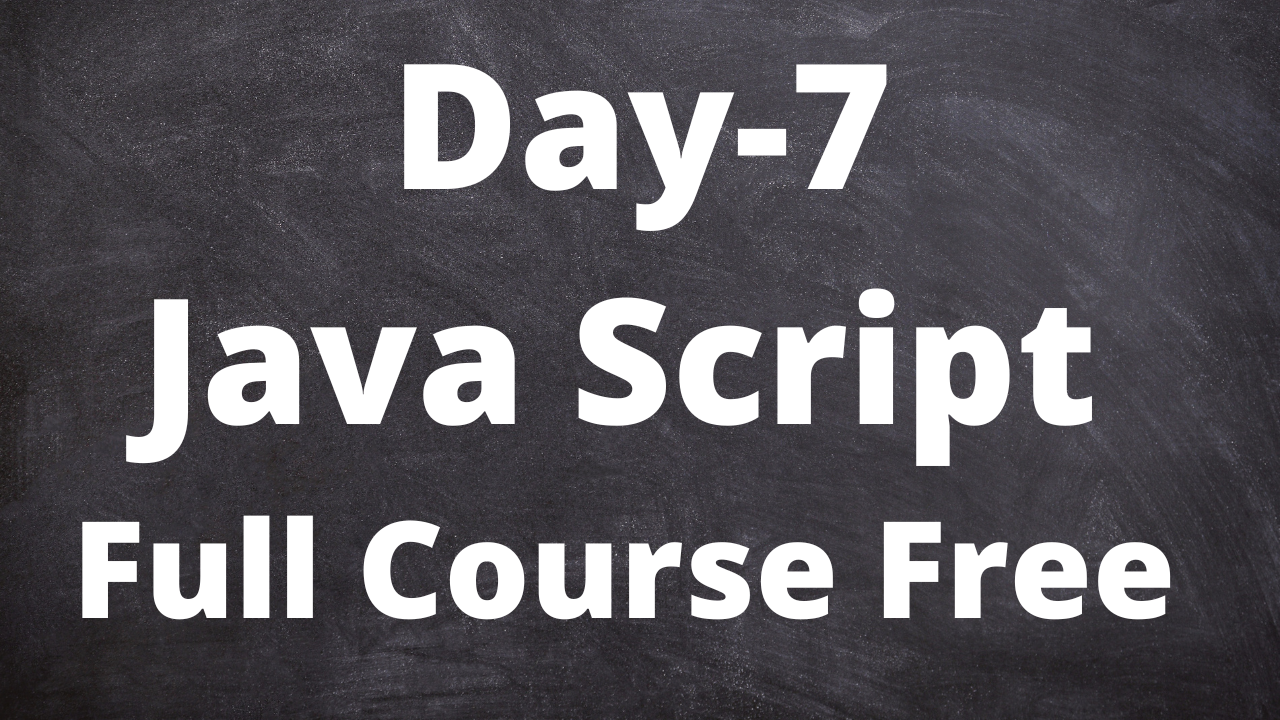
JavaScript Arrays:
Arrays store multiple values in a single variable.
var courses = new Array (“HTML”, “CSS”, “JS”);
This syntax declares an Array named Courses,
which store three values, or elements.
Accessing an Array: You refer to an Array element by referring to the index number written in square, brackets.
var courses = new Array(“HTML”, “CSS”, “JS”);
var courses = courses[0]; //HTML
courses[1] = “C++” //change the second element
Creating Arrays: JavaScrit arrays are dynamic, so you can declare an array and not pass any arguments with the Array() Constructor you can then add the elements dynamically.
Var Courses = newArray();
courses[0] = “HTML”;
courses[1] = “CSS”;
courses[2] = “JS”;
courses[3] = “C++”;
Array Literal: for simplicity, readability, and execution speed
var courses = [“HTML”, “CSS”, “JS”];
document.write(courses.length);
Combining Arrays:
JavaScript concat() method allows you to join arrays and create an entirely new Array
Example:
var c1 = [“HTML”,”CSS”];
var c2 = [“JS”,”C++”];
var courses = c1.concat(c2);
The courses Array that result Contains 4 elements (HTML, CSS, JS, C++)
Associative Arrays :
Example:
var person = []; //empty array
person[“name”] = “billo”;
person[“age”] = “35”;
document.write(person[“age”]);
Now, person is treated as an object, instead of being an array.
The named indexes “name” and “age” become properties of the person object
Math Object in JavaScript:
The Math object allows you to perform mathematical tasks.
document.write (Math.PI);
Note: Math has no Constructor there’s no need to create a math object first.
Math Object Methods:
The Math object contains a number of Methods that are used for calculations.
calculate the Square root:
var number = Math.sqrt(4);
document.write(number);
Note: To get a random number between 1-10, use Math.random()
which gives you a number between 0-1 then take Math.ceil() from it: (Math.random()*10).
Math object: lets create a program that will ask the user to input a number and alert its square root.
var n = prompt(“Enter a number” , ” * “);
var answer = Math.sqrt(n);
alert (“The Square root of ” +n +”is” + answer);
Note: Math is a handy object. You can save a lot of time using Math, instead of writing your own functions every time.
Set Interval:
The SetInterval() method calls a function or evaluates an expression at specified intervals (in milliseconds). it will continue calling the function until clear Interval() is called or the window is closed
function MyAlert(){
alert(“Hi”);
}
SetInterval(myAlert,3000);
This will call the MyAlert function every 3 seconds(1000 ms = 1 second)
write the name of the function without parentheses when passing into the SetInterval method.
Date Object in JavaScript:
Date object enables us to work with dates a date consists of a year, a month, a date, an hour, a minute, a second, and milliseconds. using newDate(), create a new date object with the Current data and time.
var d= new Date(); //d stores the current data and time
The other ways to initialize dates allow for the creating of new date objects from the specified date and time.
new Date(milliseconds)
new date (dateString)
new Date (year, month, day, hours, minutes, seconds, milliseconds)
Note: JavaScript dates are calculated in milliseconds from 01 January 1970 00:00:00 Vniversul time(UTC). One day Contains 86,400,000 milliseconds.
Example:
//Fri Jan 02 1970 00:00:00
var d1 = new Date(86400000)
//Fri Jan 02 2015 10:42:00
var d2 = new Date(“January 2, 2015 10:42:00”);
//sat Jan //1988 // 11:42:00
var d3 = new Date (88,5,11,11,42,0,0);
Note: JavaScript count month from 0to11. January is 0, and December is 11
Date object are static, rather than dynamic. the computer time is ticking, but date objects do not change Once Created.
Let’s Create a program that prints the current time to the browser once every second.
function printTime(){
var d = new Date();
var hours = d.getHours();
var min = d.getMinutes();
var secs = d.getSeconds();
document.body.innerHTML = hours+”:” +ins+”;”+secs; }
setInterval(printTime,1000);
Note: The inner HTML property sets or returns the HTML Content of an element.
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all