JavaScript Functions
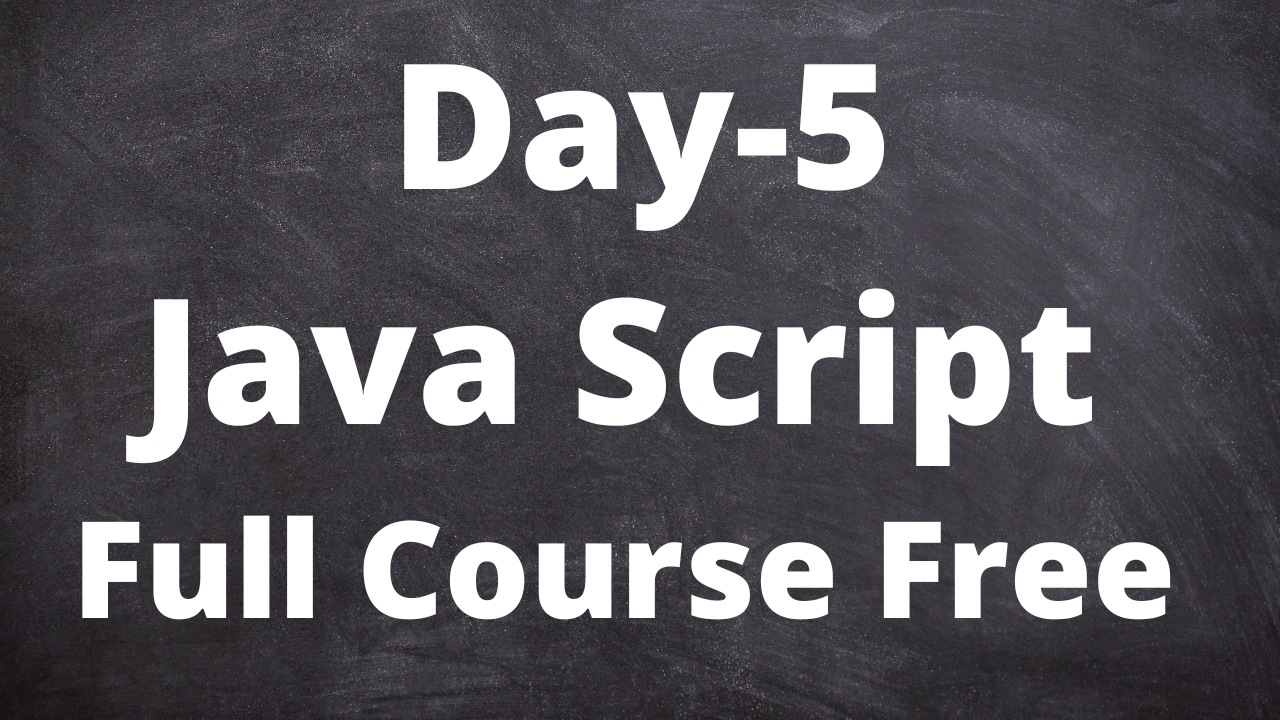
JavaScript Functions
Java Script function is a block of code designed to perform a particular task. the main advantage of using functions code reuse define the code once and use it many times.
function name (){
// code to be executed
}
Note: function names can contain letters, digits, underscores, and dollar signs (same rules as variables)
Calling a Function
To execute the function, you need to call it. to call a function, start with the name of the function, then follow it with the arguments in parentheses.
Example:
function myFunction(){
alert(“Calling a function !”)
}
myFunction();
Note: always number the ends of the calling function with; a semicolon.
Function myFunction(){
alert(“Alert box !);
}
myFunction();
// “Alert box !”
// “Some other code”
myFunction();
// “Alert box !”
Note: you can also call a function using this syntax: myFunction.calling the difference is that when calling in this way, you are passing the ‘this ‘ keyword to a function. you will learn about it later
Function Parameters:
A function can take Parameters.
FunctionName(Pr1, Pre2, Pre3){
//code
}
Using Parameters:
function sayHello(name){
ALEART(“Hi,” + name);
}
sayHello(“Billo”)
Output: Hi Billo
Multiple Parameters:
we can define multiple parameters for a function by comma-separating them
function myFunc{
//——-
}
Example:
function test(x,y){
if(x>y){
document.write(x);
}
else{
document.write(y);
}
}
test(5,8);
Output: 8
Example:
function sayHello(name,age){
document.write(name+”is”+age+”years old.”);
}
sayHello(“Billo”35)
Output: Billo is 35 years old.
Function Return:
A function can have an optional return statement. it is used to return a value from the function.
This statement is useful when making evaluations that require a result
Example:
function myFunction(b,g){
return b*g;
}
var x = myfunction(35,30);
//Return value will end up in x
Output: 1050
Example: function addNumber(b,g){
var z = b+g;
return z;
}
document.write(addNumber(40,2));
JavaScript Alert Box:
JavaScript offers three types of popup boxes, the Alert, Prompt, and Confirm boxes.
Alert Example:
alert(“Do you really want to leave this page ?”);
output: Do you really want to leave this page?
Ok
To display line break: alert (“Hello\nHow are you?”);
output: Hello
How are you?
Ok
Prompt Box: Prompt box is often used to have the user input
Example:
var users = prompt(“Please enter your name “);
alert(user);
Confirm Box: A confirm box is after used to have the user verify or accept something.
Example:
var result = confirm (“Do you really want to leave this page? “);
if(result == true){
alert (“Thanks for visiting”);
}
else{
alert(“Thanks for staying with us”);
}
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all