SQL: VIEW
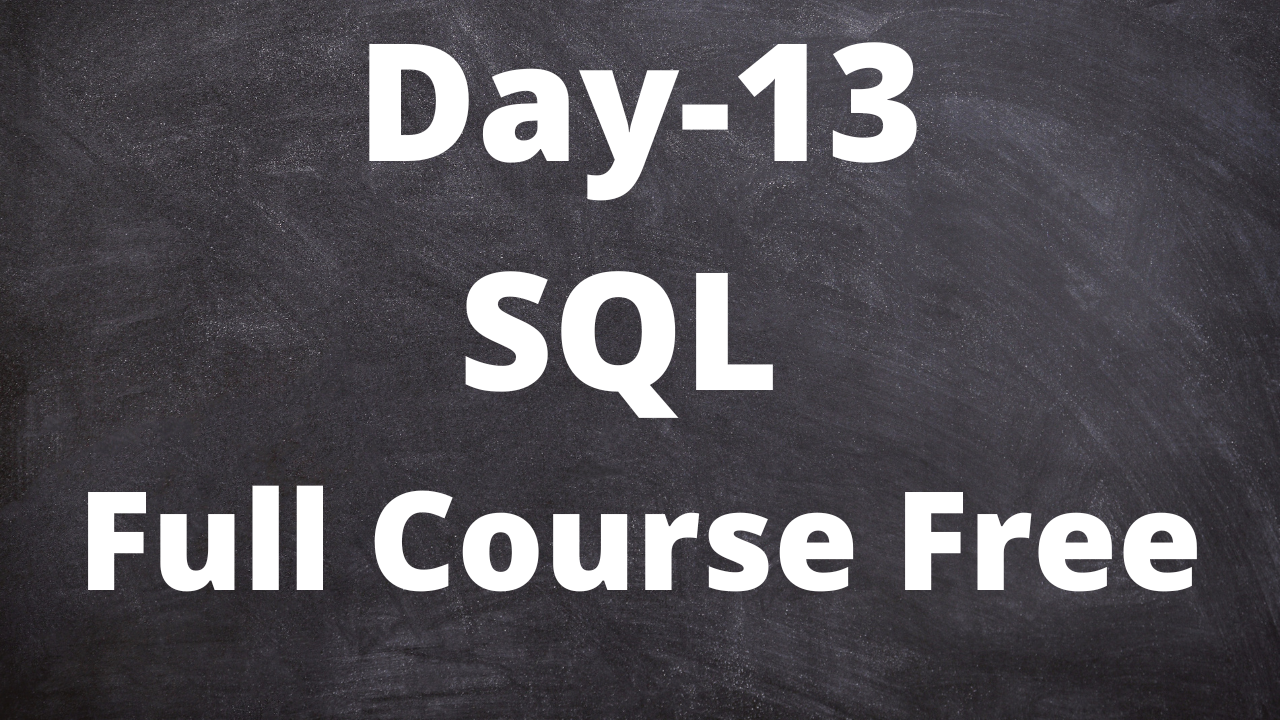
SQL: VIEW
In SQL, a VIEW is a virtual table that is derived from the result of a query. It is created based on an existing table or multiple tables, and it can be used like a regular table in SQL queries. Views provide a way to simplify complex queries, encapsulate business logic, and enhance data security by controlling access to specific columns or rows. Here’s an explanation of views with an example:
Let’s say we have a table called “employees” with the following structure:
CREATE TABLE employees (
id INT,
name VARCHAR(50),
age INT,
department VARCHAR(50)
);
Now, we can create a view that displays only the name and department of employees who belong to the “Sales” department:
CREATE VIEW sales_employees AS
SELECT name, department
FROM employees
WHERE department = 'Sales';
In this example, we created a view named “sales_employees” that selects the name and department columns from the “employees” table, but only for employees in the “Sales” department. The view definition acts as a saved query.
Once the view is created, you can use it in queries just like a regular table:
SELECT * FROM sales_employees;
This query will retrieve the name and department of employees who belong to the “Sales” department, but it’s executed against the view rather than directly against the base table.
Views can also be used to simplify complex queries by joining multiple tables, aggregating data, or applying filters. They provide a convenient way to encapsulate commonly used logic and make query operations more efficient.
It’s important to note that views do not store any data themselves; they are just stored queries that are dynamically executed whenever the view is referenced in a query. Any changes made to the underlying tables will be reflected in the view’s results.
Additionally, views can be used to restrict access to sensitive data. For example, you can create a view that only exposes specific columns to certain users or groups, hiding the rest of the table’s data.
Overall, views in SQL offer a powerful way to organize and manipulate data, simplify queries, and enhance data security and access control.
SQL: Creating Views
To create a view in SQL, you can use the CREATE VIEW statement. Here’s the general syntax:
CREATE VIEW view_name AS
SELECT column1, column2, ...
FROM table_name
WHERE condition;
Let’s illustrate the process of creating a view with an example:
Suppose we have a table called “orders” that stores information about customer orders, including order ID, customer ID, and order date:
CREATE TABLE orders (
order_id INT,
customer_id INT,
order_date DATE
);
Now, let’s create a view that shows the order details for orders placed in the current year:
CREATE VIEW current_year_orders AS
SELECT order_id, customer_id, order_date
FROM orders
WHERE YEAR(order_date) = YEAR(CURRENT_DATE());
In this example, we created a view named “current_year_orders” that selects the order_id, customer_id, and order_date columns from the “orders” table. The view definition includes a condition in the WHERE clause to filter the orders based on the current year.
Once the view is created, you can query it just like you would a regular table:
SELECT * FROM current_year_orders;
This query will retrieve the order details for orders placed in the current year, as defined by the view.
It’s important to note that views don’t store any data themselves; they are simply stored queries. Therefore, any changes made to the underlying tables will be reflected in the view’s results. Additionally, views can be used to simplify complex queries, join multiple tables, perform aggregations, or apply filters.
Remember to consider the database system you’re using, as syntax and supported features may vary slightly.
SQL: Updating a VIEW
In SQL, views are generally used for querying data rather than updating or modifying it. By default, you cannot directly update a view that is based on multiple tables or complex logic. However, you can update a view under certain conditions by using the WITH CHECK OPTION
clause. Let’s go through an example to understand this concept.
Suppose we have two tables: “employees” and “departments”.
- The “employees” table has the following structure:
CREATE TABLE employees (
employee_id INT,
name VARCHAR(50),
department_id INT
);
It contains information about employees, including their IDs, names, and department IDs.
- The “departments” table has the following structure:
CREATE TABLE departments (
department_id INT,
name VARCHAR(50)
);
It stores information about departments, including their IDs and names.
Now, let’s create a view that displays the names of employees and their corresponding department names.
CREATE VIEW employee_department AS
SELECT employees.name AS employee_name, departments.name AS department_name
FROM employees
JOIN departments ON employees.department_id = departments.department_id;
In this example, we created a view named “employee_department” that joins the “employees” and “departments” tables based on the department ID. The view selects the employee names and their corresponding department names.
You can query the view as follows:
SELECT * FROM employee_department;
Now, let’s say you want to update the name of an employee through the view. To achieve this, you can use the WITH CHECK OPTION
clause while creating the view.
CREATE VIEW employee_department AS
SELECT employees.name AS employee_name, departments.name AS department_name
FROM employees
JOIN departments ON employees.department_id = departments.department_id
WITH CHECK OPTION;
The WITH CHECK OPTION
ensures that any updates performed through the view adhere to the view’s criteria. However, note that the ability to update a view depends on various factors, including the complexity of the view’s definition and the underlying tables.
To update a view, you need to perform the update on the underlying tables directly rather than updating the view itself. The view will reflect the updated data when queried.
Keep in mind that the ability to update a view is limited and depends on the specific database management system and the characteristics of the view. It’s always recommended to consult the documentation of your database system for the most accurate and up-to-date information regarding updating views.
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all