JavaScript Replacing Elements
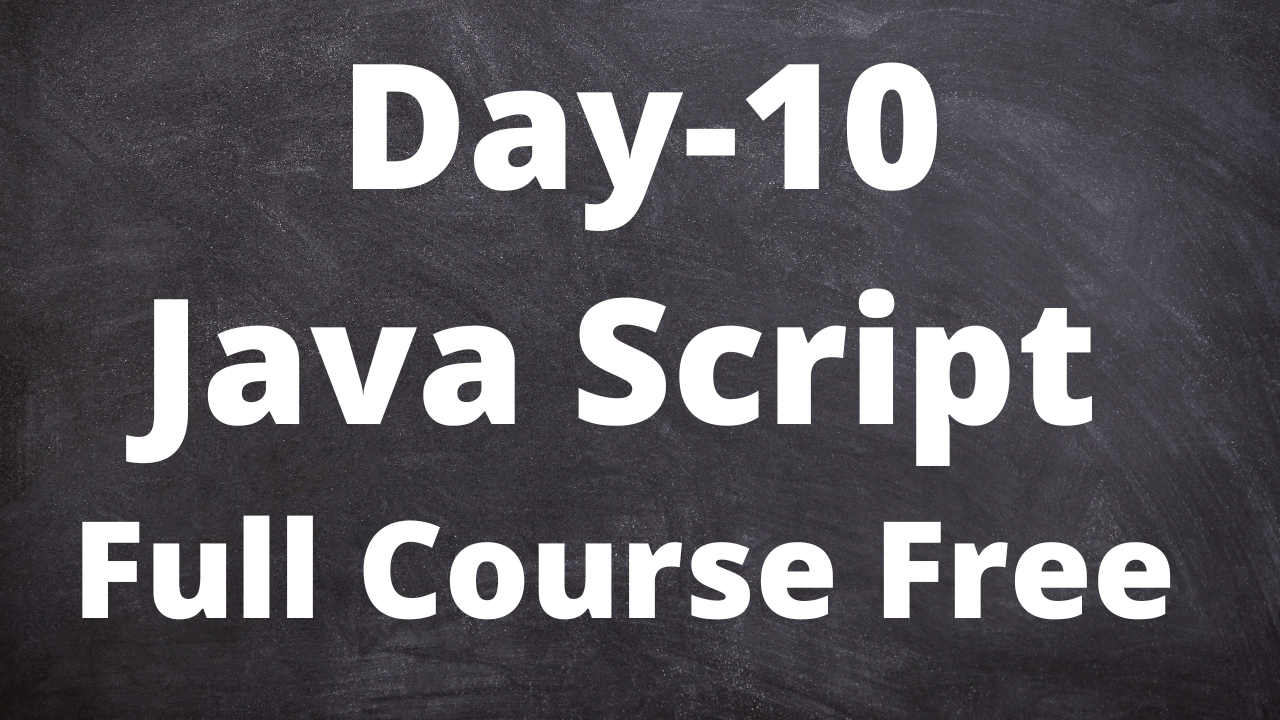
JavaScript Replacing Elements
To replace an HTML element, the element.replaceChild(new Node, old Node) method is used.
Example:
<div id = “demo”>
<P id= “P1”> this is a paragraph </p>
<P id= “P2”> this is another paragraph </p>
</div>
<Script>
var P = document.CreateElement(“P”);
var node = document.CreateTextNode(“This is new”);
P.appendChild(node);
var Parent = document.getElementById(“demo”);
var child = document.getElementById(“P1”)
Parent.replacechild(P,child);
</Script>
Note: The code above Creates a new Paragraph element that replaces the existing P1 Paragraph.
Animations:
Now we know How to select and chang DOM element lets create a simple HTML Page with a box element that will be animated using JS
<Style>
#Container{
width: 200px;
height: 200px;
background: green;
position: relative;
}
#Box{
width: 50px;
height: 50px;
background: red;
position: absolute;
}
</Style>
< div id = “container”>
<div id = “box”> </div>
</div>
Animations Interval
we can achive this by using the setInteraval() method,
var t = SetInterval(move,500);
This code creates a timer that calls a move() function every 500 milliseconds. Now we need to define the move() function. that change position of box.
//String Position
var pos = 0;
//our box element
var box = document.getElementById(“Box”);
function move(){
pos +=1;
box.style.left = pos+”px”; //px = pixels
}
Example: JavaScript Animation
var pos = 0;
//ourbox element
var box = document.getElementById(“box”);
var t = setInterval(move,10);
function move(){
if(pos >=150){
clearInterval(t);
}
else{
pos +=1;
box.style.left = post+”px”;
}
}
Event:
JavaScript code that executes when an event occurs, such as when user click an HTML element, move the mouse or Submits a form.
Note: Corresponding events can be added to HTML element as attributes
Example:
<p on click = “show()”> some text </p>
Handling Events:
Example:
button Onclick = “show()” Click Me</button>
<Script>
function show(){
alert(“Hi There ..!!”);
}
</Script>
Example: var x = document.getElementById(“demo”);
x.Onclick = function(){
document.body.innerHTML = Date();
}
Note: we can attach events to all most all HTML elements.
Events:
The Onload and Onunload events are triggered when the user enters or leaves the page. These can be useful when performing actions after the page is loaded.
<body Onload = “do Something()”>
Similarly, the window.Onload event can be used to run code after the whole page is loaded.
window.Onload = function(){
//soe code
}
Onchange event is mostly used on text boxes. the event handler get called when the text inside the text box changes and focus is lost from the element.
<input type = “text” id = “name” Onchange = “change()”>
<Script>
function change(){
var x =document.getElementById(“name”);
x.value= x.value.toUpperCase();
}
</Script>
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all