JavaScript Working With DOM
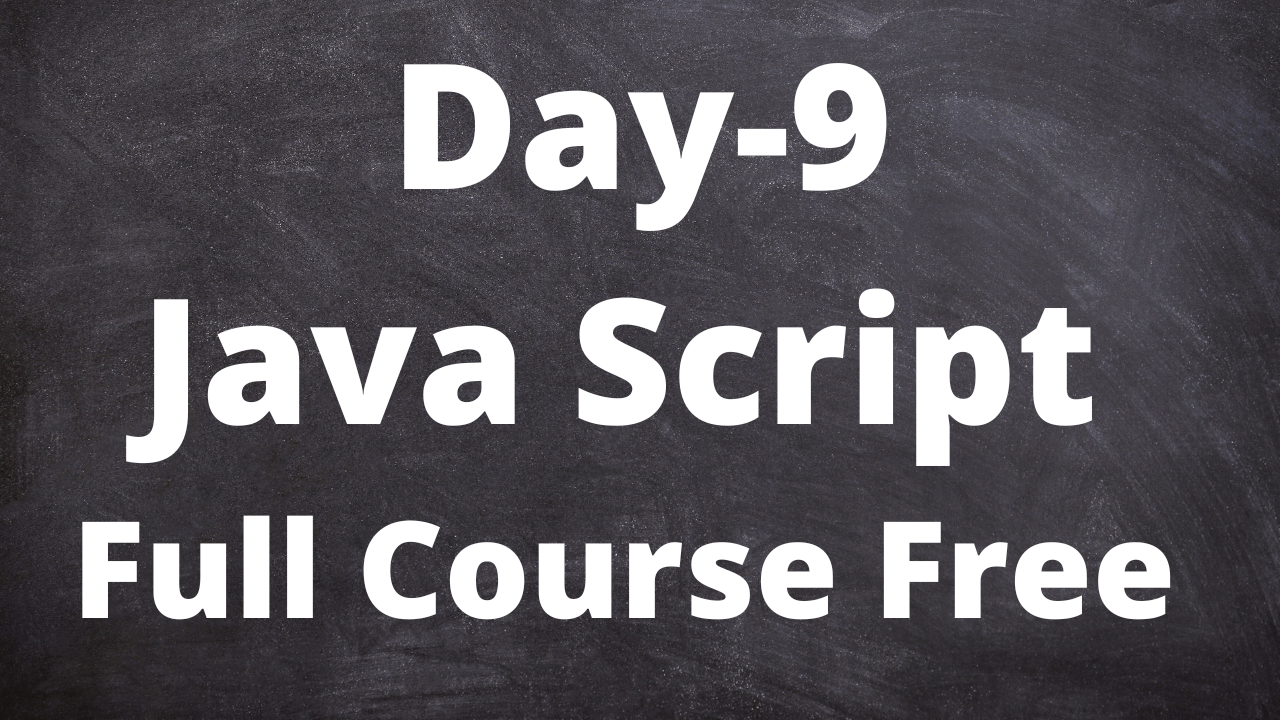
JavaScript Working With DOM
element.childNodes //returns an array of an element child nodes.
element.firstchild //returns the first child node of an element
element.lastchild //returns the last child node of an element
element.haschildNodes //returns true if an element has any child node other wise false
element.previousSibling // return the previous node at the same tree level.
element.ParentNode // return the parent node at an element
<HTML>
<body>
<div id = “demo”>
<P>some text </P>
<P>some other text </P>
</div>
<Script>
var a = document.getElementById(“demo”);
var arr = a.childNodes;
for(var x = 0; x<arr,length; x++){
arr[x].innerHTML = “new text”;}
</Script>
</body>
</HTML>
Changing Attributes in JavaScript
we can change text element of an element using the inner HTML Property
<img id = “myimg” src = “orange.png” alt = ” “/>
<Script>
var el = document.getElementById(“myimg”);
el.src = “apple.png”;
</Script>
WE CAN CHANGE href attribute of a link
<a href = “HTTP://www.boomitechie.com”> somelink</a>
<script>
var el = document.getElemensByTagName(“a”);
el[0].herf = “https:www.boomitechie.com”;
</script>
Changing Style: All style attributes can be accessed using the style object of the element.
<div id = “demo” style = “width:200px> some text </div>
<script>
var x = document.getElementById(“demo”);
x.style.color = “6600FF”;
X.style.with = “100 px”;
</Script>
The code above the text color and width of the div element
Creating Elements
Use the following method to create new nodes:
element.cloneNode()
clones an element and returns the resulting node.
document.CreateElement //create new element node
document.CreateTextNode //create new element node
Example:
var node = document.CreateTextNode(“some new Text”);
element.append child(newNode) // add a new child node to the element as the last child node
element.insertBefore(node1,node2) insert node1 as child before node 2
Example:
<div id = “demo”> some content </div>
<Script>
var p = document.createElement(“P”);
var node = document.CreateTextNode(“some new text”);
P.appendChild(node);
var div = document.getElementById(“demo”);
div.appendChild(P);
</Script>
Removing Elements: RemoveChild(node)method.
<div id = “demo”>
<P id = “P1”> this is paragraph </P>
<P id = “P2”> this is another paragraph </P>
</div>
<Script>
varParent = document.getElementById(“demo”);
var child = document.getElementById(“P1”);
Parent.removeChild(child);
</Script>
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all