SQL: CONCAT Function with Example
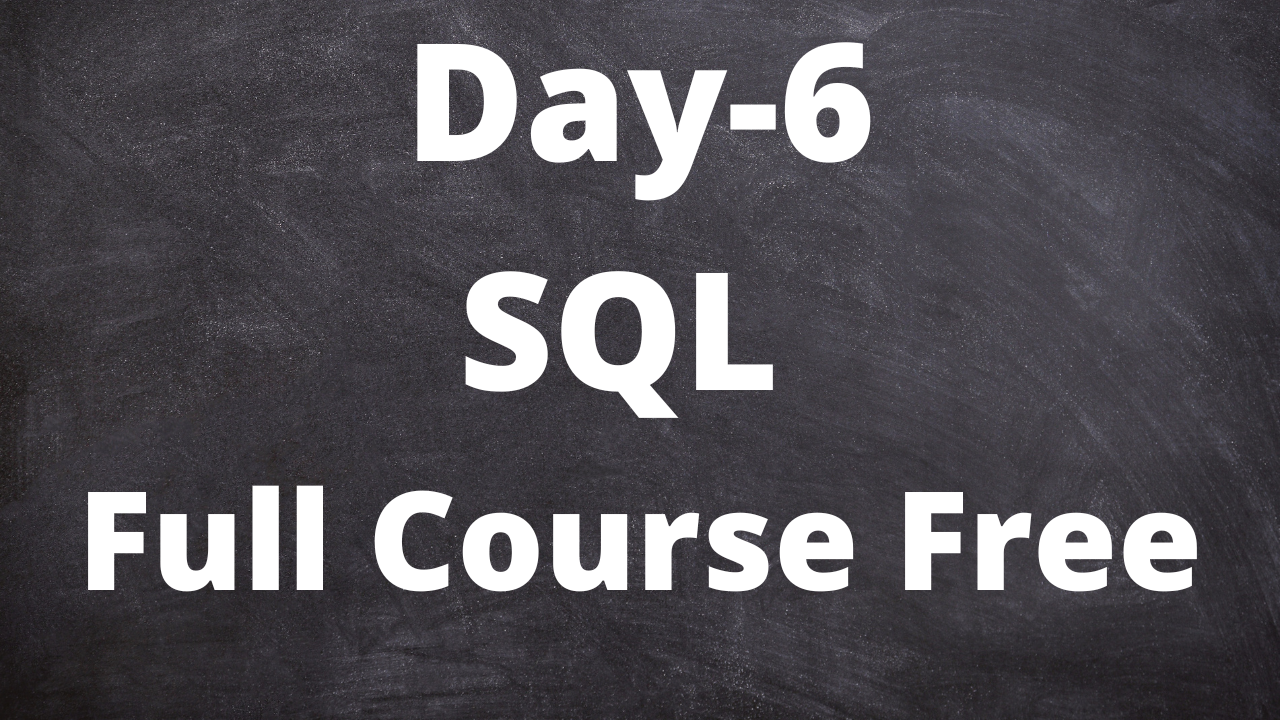
SQL: CONCAT Function with Example
In SQL, the CONCAT function is used to concatenate or join multiple strings together. It allows you to combine two or more strings into a single string. Here’s an explanation with an example:
Consider a table named “employees” with columns: first_name, last_name. Here’s a sample data:
+------------+-----------+
| first_name | last_name |
+------------+-----------+
| John | Smith |
| Jane | Doe |
| Alex | Johnson |
| Lisa | Brown |
+------------+-----------+
To concatenate the “first_name” and “last_name” columns into a single string, you can use the CONCAT function in your SQL query. Here’s an example:
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM employees;
Output:
+--------------+
| full_name |
+--------------+
| John Smith |
| Jane Doe |
| Alex Johnson |
| Lisa Brown |
+--------------+
In this example, the SELECT statement uses the CONCAT function to concatenate the values of the “first_name” and “last_name” columns. The space character (‘ ‘) is also included within the CONCAT function to add a space between the first name and last name in the resulting concatenated string. The result is displayed as a new column named “full_name”.
The CONCAT function takes multiple string arguments and concatenates them in the specified order. You can include additional strings or literals within the CONCAT function, such as additional characters or separators.
It’s important to note that the behavior of the CONCAT function may vary slightly depending on the database management system (DBMS) you are using. Some DBMSs may have different syntax or alternative functions for string concatenation, such as the “+” operator in some systems.
The CONCAT function is a powerful tool for manipulating strings in SQL queries, allowing you to combine and format strings to meet your specific requirements.
SQL: AS Keyword with Example
In SQL, the AS keyword is used to provide an alias or a temporary name for a column or a table in the result set of a query. It allows you to assign a new name to a column or a table that can be used in the query output or in subsequent parts of the query. Here’s an explanation with an example:
- Alias for a Column:
Consider a table named “employees” with columns: first_name, last_name. Here’s a sample data:
+------------+-----------+
| first_name | last_name |
+------------+-----------+
| John | Smith |
| Jane | Doe |
| Alex | Johnson |
| Lisa | Brown |
+------------+-----------+
To assign an alias to a column, you can use the AS keyword in your SQL query. Here’s an example:
SELECT first_name AS fname, last_name AS lname FROM employees;
Output:
+--------+--------+
| fname | lname |
+--------+--------+
| John | Smith |
| Jane | Doe |
| Alex | Johnson|
| Lisa | Brown |
+--------+--------+
In this example, the SELECT statement renames the “first_name” column to “fname” and the “last_name” column to “lname” using the AS keyword. The aliases are used as the column names in the resulting output.
- Alias for a Table:
Consider two tables: “employees” and “departments”. Here’s a sample data: “employees” table:
+----+------------+-----------+
| id | first_name | last_name |
+----+------------+-----------+
| 1 | John | Smith |
| 2 | Jane | Doe |
+----+------------+-----------+
“departments” table:
+----+------------+
| id | department |
+----+------------+
| 1 | Sales |
| 2 | HR |
+----+------------+
To assign an alias to a table, you can use the AS keyword in your SQL query. Here’s an example:
SELECT emp.first_name, emp.last_name, dep.department
FROM employees AS emp
JOIN departments AS dep ON emp.id = dep.id;
Output:
+------------+-----------+------------+
| first_name | last_name | department |
+------------+-----------+------------+
| John | Smith | Sales |
| Jane | Doe | HR |
+------------+-----------+------------+
In this example, the SELECT statement assigns aliases “emp” and “dep” to the “employees” and “departments” tables, respectively, using the AS keyword. The aliases are used to reference the tables and their columns in the query.
The AS keyword provides a way to assign temporary names or aliases to columns or tables in SQL queries. It can make query results more readable and allow for clearer referencing of columns or tables in complex queries involving multiple tables or calculated columns.
SQL: Arithmetic Operators with Example
In SQL, arithmetic operators are used to perform mathematical operations on numeric values within SQL queries. These operators allow you to perform addition, subtraction, multiplication, division, and more. Here’s an explanation of the arithmetic operators in SQL with examples:
- Addition Operator (+):
The addition operator is used to perform addition between two or more numeric values. Example:
SELECT 10 + 5;
Output:
15
- Subtraction Operator (-):
The subtraction operator is used to perform Subtraction between two numeric values. Example:
SELECT 20 - 8;
Output:
12
- Multiplication Operator (*):
The multiplication operator is used to perform multiplication between two numeric values. Example:
SELECT 6 * 4;
Output:
24
- Division Operator (/):
The division operator is used to perform division between two numeric values. Example:
SELECT 20 / 5;
Output:
4
- Modulus Operator (%):
The modulus operator returns the remainder of a division operation between two numeric values. Example:
SELECT 17 % 5;
Output:
2
These are some basic arithmetic operators available in SQL. They allow you to perform mathematical calculations within SQL queries. Arithmetic operators can be used with literal values, column values, or expressions involving multiple operators.
It’s important to note that when performing arithmetic operations on columns, the data types of the columns should be compatible with the specific operation being performed. Additionally, parentheses can be used to control the order of operations, just like in regular mathematical expressions.
By utilizing arithmetic operators effectively, you can perform calculations, create derived columns, and manipulate numeric values in SQL queries.
SQL: UPPER Function with Example
In SQL, the UPPER function is used to convert a string to uppercase. It is a string function that takes a string as input and returns the uppercase version of that string. Here’s an explanation with an example:
Consider a table named “employees” with a column named “first_name”. Here’s a sample data:
+------------+
| first_name |
+------------+
| John |
| Jane |
| Alex |
| Lisa |
+------------+
To convert the “first_name” column values to uppercase, you can use the UPPER function in your SQL query. Here’s an example:
SELECT UPPER(first_name) AS upper_name FROM employees;
Output:
+------------+
| upper_name |
+------------+
| JOHN |
| JANE |
| ALEX |
| LISA |
+------------+
In this example, the SELECT statement uses the UPPER function to convert the “first_name” column values to uppercase. The resulting uppercase strings are displayed as a new column named “upper_name” in the output.
The UPPER function is commonly used when you want to standardize or manipulate text data by converting it to uppercase. It can be useful for formatting, comparison, or consistency purposes in SQL queries.
It’s important to note that the behavior of the UPPER function may vary slightly depending on the database management system (DBMS) you are using. Some DBMSs may have alternative functions or collation settings that affect string case conversions.
The UPPER function is a powerful tool for transforming string values to uppercase in SQL queries, allowing you to perform case-insensitive comparisons, standardize formatting, or modify text data according to your specific needs.
SQL: SQRT and AVG with Example
In SQL, the SQRT function is used to calculate the square root of a numeric value, while the AVG function is used to calculate the average value of a set of numeric values. Here’s an explanation of each function with examples:
- SQRT Function:
The SQRT function calculates the square root of a given numeric value. It takes one argument, which is the numeric value for which you want to find the square root. Example:
SELECT SQRT(16) AS square_root;
Output:
+--------------+
| square_root |
+--------------+
| 4.0 |
+--------------+
In this example, the SELECT statement uses the SQRT function to calculate the square root of 16. The result, 4.0, is displayed as “square_root” in the output.
- AVG Function:
The AVG function calculates the average value of a set of numeric values. It takes a column or an expression as an argument and returns the average value. Example:
Consider a table named “employees” with a column named “salary”. Here’s a sample data:
+--------+
| salary |
+--------+
| 5000 |
| 6000 |
| 4500 |
| 7000 |
+--------+
To calculate the average salary of the employees, you can use the AVG function in your SQL query. Here’s an example:
SELECT AVG(salary) AS average_salary FROM employees;
Output:
+----------------+
| average_salary |
+----------------+
| 5625.00 |
+----------------+
In this example, the SELECT statement uses the AVG function to calculate the average value of the “salary” column. The resulting average salary, 5625.00, is displayed as “average_salary” in the output.
The SQRT and AVG functions are useful for performing mathematical calculations and aggregations on numeric values within SQL queries. They allow you to calculate square roots and average values, respectively, providing valuable insights and facilitating data analysis.
SQL: SUM FUNCTION with Example
In SQL, the SUM function is used to calculate the sum of a set of numeric values. It takes a column or an expression as an argument and returns the total sum of the values. Here’s an explanation of the SUM function with an example:
Consider a table named “sales” with a column named “amount”. Here’s a sample data:
+--------+
| amount |
+--------+
| 100 |
| 150 |
| 200 |
| 75 |
+--------+
To calculate the total sum of the “amount” column, you can use the SUM function in your SQL query. Here’s an example:
SELECT SUM(amount) AS total_amount FROM sales;
Output:
+--------------+
| total_amount |
+--------------+
| 525 |
+--------------+
In this example, the SELECT statement uses the SUM function to calculate the sum of the “amount” column. The resulting total sum, 525, is displayed as “total_amount” in the output.
The SUM function is commonly used in SQL when you need to aggregate or summarize numeric data, such as sales figures, revenue, quantities, or any other measurable values. It allows you to quickly obtain the sum of a column’s values, providing valuable insights and facilitating data analysis.
It’s important to note that the SUM function operates on numeric data types and ignores any NULL values within the column. Additionally, you can also use the SUM function in conjunction with the GROUP BY clause to calculate sums for specific groups within your data.
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all