SQL multiple queries or commands with example
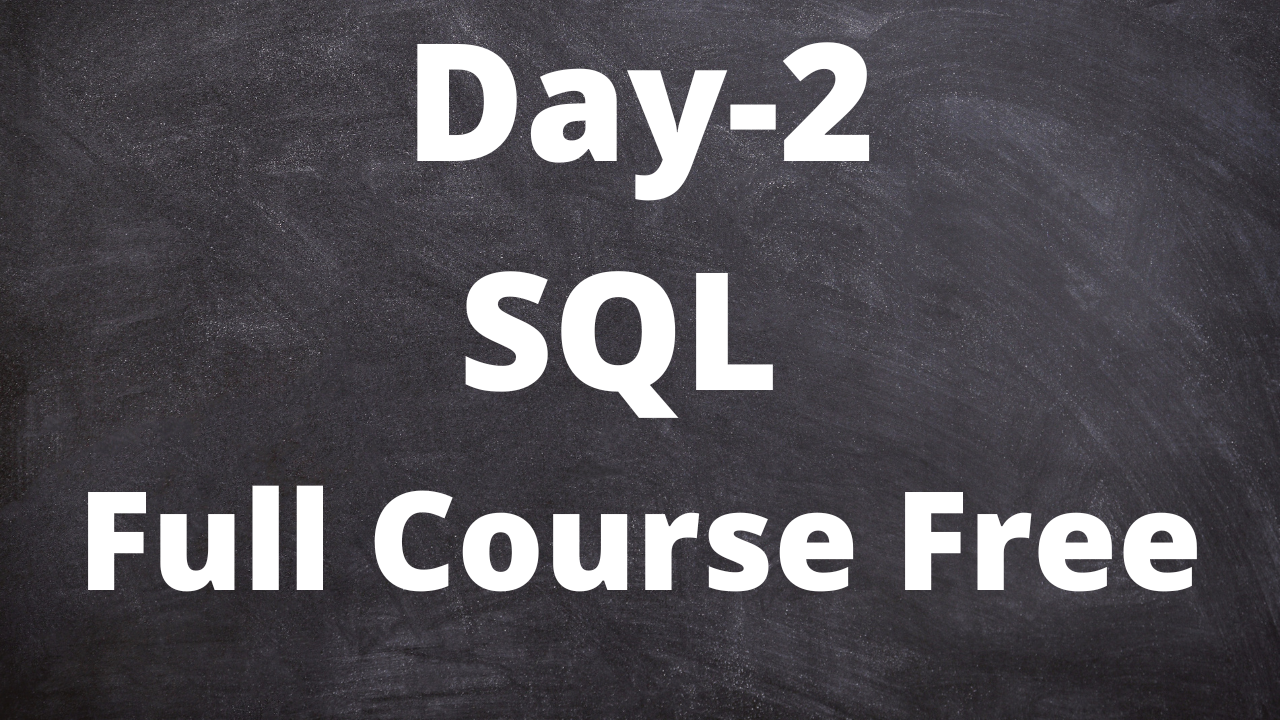
SQL multiple queries or commands with example
When it comes to executing multiple queries or commands in SQL, most database management systems do not support directly executing multiple statements in a single query. However, you can execute multiple queries or commands sequentially by separating them with semicolons and executing each query/command individually. Here’s an example:
sqlCopy code-- First query
SELECT * FROM employees;
-- Second query
UPDATE employees SET salary = salary * 1.1 WHERE department = 'Sales';
-- Third query
INSERT INTO new_employees (first_name, last_name) VALUES ('John', 'Doe');
In this example, we have three separate queries or commands: a SELECT statement to retrieve data from the “employees” table, an UPDATE statement to modify salary values based on a condition, and an INSERT statement to add a new employee to the “new_employees” table.
To execute these queries or commands, you would typically send them to the database server one by one using your preferred database client or programming language. Each query/command would be executed separately, and you would handle the results or any errors accordingly.
Please note that executing multiple queries or commands in a single call is generally not recommended due to security risks such as SQL injection. It’s important to sanitize and validate user input or use prepared statements to prevent unauthorized access or malicious attacks.
Is SQL Case Sensitive? Here is Explained with Example
The case sensitivity in SQL depends on the specific database management system (DBMS) being used. In general, SQL is not case-sensitive for keywords and identifiers, but it can be case-sensitive for string values depending on the collation settings of the database.
Let’s consider an example using the MySQL DBMS, which is case-insensitive for keywords and identifiers by default:
-- Creating a table
CREATE TABLE employees (
id INT,
first_name VARCHAR(50),
last_name VARCHAR(50)
);
-- Inserting data into the table
INSERT INTO employees (id, first_name, last_name)
VALUES (1, 'John', 'Smith');
-- Querying the data
SELECT * FROM employees;
In this example, we created a table named “employees” with columns for ID, first name, and last name. We inserted a record with the values 1, ‘John’, and ‘Smith’ into the table. Finally, we queried the data using the SELECT statement.
Now, let’s consider a case-sensitive scenario using the PostgreSQL DBMS, which is case-sensitive for both keywords/identifiers and string values by default:
-- Creating a table
CREATE TABLE "employees" (
"ID" INT,
"FirstName" VARCHAR(50),
"LastName" VARCHAR(50)
);
-- Inserting data into the table
INSERT INTO "employees" ("ID", "FirstName", "LastName")
VALUES (1, 'John', 'Smith');
-- Querying the data
SELECT * FROM "employees";
In this example, we created a table named “employees” with columns for “ID”, “FirstName”, and “LastName”. Notice that the column names and table name are enclosed in double quotes to preserve the case sensitivity. We inserted a record and queried the data using the SELECT statement, making sure to use the exact case-sensitive column and table names.
It’s important to note that the behavior of case sensitivity can vary among different DBMSs and can also be influenced by the collation settings of the database. It’s always recommended to refer to the specific documentation or resources of the DBMS you are working with to understand its case sensitivity rules and behavior.
what are SQL syntax rules, show the example
SQL follows certain syntax rules that govern how queries and commands are structured. Here are some key SQL syntax rules, along with examples:
- Statements should be terminated by a semicolon (;):
SELECT * FROM employees;
- Keywords and identifiers are case-insensitive, but it is common to use uppercase for keywords and lowercase for identifiers:
SELECT column1 FROM Employees;
- Whitespace and line breaks can be used for readability, and multiple spaces are treated as one:
SELECT column1, column2
FROM employees
WHERE age > 25;
- Single-line and multi-line comments can be used to document code:
-- This is a single-line comment
/*
This is a multi-line comment.
It can span multiple lines.
*/
- SQL string values should be enclosed in single quotes (‘ ‘):
SELECT * FROM employees WHERE first_name = 'John';
- Column and table names with spaces or special characters should be enclosed in double quotes (” “):
SELECT "column name" FROM "table name";
- Wildcards, such as asterisk (*), can be used to select all columns:
SELECT * FROM employees;
- SQL is generally not sensitive to whitespace, so you can use it to improve readability:
SELECT column1,
column2
FROM employees
WHERE age > 25;
- SQL clauses, such as SELECT, FROM, WHERE, etc., are usually written in uppercase:
SELECT column1
FROM employees
WHERE age > 25;
- SQL allows you to use operators, such as =, <, >, AND, OR, etc., to perform comparisons and logical operations:
sql SELECT * FROM employees WHERE age > 25 AND department = 'Sales';
These are some of the common SQL syntax rules. It’s important to note that different database management systems (DBMS) may have some variations in syntax and additional features. It’s always recommended to refer to the documentation or resources specific to your DBMS for accurate syntax details and examples.
SQL: How to select multiples columns with example
To select multiple columns in SQL, you can simply list the column names separated by commas in the SELECT statement. Here’s an example:
Consider a table named “employees” with columns: id, first_name, last_name, and age. Here’s a sample data:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 1 | John | Smith | 28 |
| 2 | Jane | Doe | 32 |
| 3 | Alex | Johnson | 24 |
| 4 | Lisa | Brown | 30 |
+----+------------+-----------+-----+
To select multiple columns from this table, you can use the following SQL query:
SELECT first_name, last_name, age FROM employees;
Output:
+------------+-----------+-----+
| first_name | last_name | age |
+------------+-----------+-----+
| John | Smith | 28 |
| Jane | Doe | 32 |
| Alex | Johnson | 24 |
| Lisa | Brown | 30 |
+------------+-----------+-----+
In this example, the SELECT statement retrieves the first_name, last_name, and age columns from the employees table. The result set will contain the values of these columns for all the rows in the table.
You can select any number of columns by listing their names separated by commas within the SELECT statement.
SQL: How to select all columns in SQL with example
To select all columns in SQL, you can use the asterisk (*) symbol in the SELECT statement. Here’s an example:
Consider a table named “employees” with columns: id, first_name, last_name, and age. Here’s a sample data:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 1 | John | Smith | 28 |
| 2 | Jane | Doe | 32 |
| 3 | Alex | Johnson | 24 |
| 4 | Lisa | Brown | 30 |
+----+------------+-----------+-----+
To select all columns from this table, you can use the following SQL query:
SELECT * FROM employees;
Output:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 1 | John | Smith | 28 |
| 2 | Jane | Doe | 32 |
| 3 | Alex | Johnson | 24 |
| 4 | Lisa | Brown | 30 |
+----+------------+-----------+-----+
In this example, the SELECT statement with the asterisk (*) selects all columns from the employees table. The result set will contain all the columns and their respective values for all the rows in the table.
Using the asterisk (*) is convenient when you want to retrieve all columns from a table without explicitly listing their names. However, be cautious when using it in production scenarios, as it may retrieve more data than necessary and impact performance. It’s generally recommended to explicitly specify the required columns for better clarity and efficiency.
SQL: distinct keyword, Explain with Example
The DISTINCT keyword in SQL is used to retrieve unique or distinct values from a column or a combination of columns in a query result set. It eliminates duplicate values and returns only the unique values. Here’s an explanation with an example:
Consider a table named “employees” with a column named “department” that stores the department names of employees. Here’s a sample data:
+-----+
| department |
+-----+
| Sales |
| HR |
| Sales |
| IT |
| HR |
+-----+
To retrieve the distinct or unique department names from this table, you can use the DISTINCT keyword in the SELECT statement, like this:
SELECT DISTINCT department FROM employees;
Output:
+-----+
| department |
+-----+
| Sales |
| HR |
| IT |
+-----+
In this example, the SELECT statement with the DISTINCT keyword retrieves only the unique values from the “department” column. It eliminates the duplicate occurrences of the department names and returns only the distinct values.
The DISTINCT keyword is useful when you want to analyze unique values or perform aggregations on unique data. It helps in obtaining a unique set of values from a specific column or a combination of columns in a result set.
It’s important to note that the DISTINCT keyword applies to all the columns specified in the SELECT statement. If you want to retrieve distinct combinations of multiple columns, you would use the DISTINCT keyword with those columns together.
SELECT DISTINCT column1, column2 FROM table_name;
This would retrieve distinct combinations of values from both “column1” and “column2”.
SQL: LIMIT keyword, Explain with Example
The LIMIT keyword in SQL is used to restrict the number of rows returned by a query. It allows you to specify the maximum number of rows to retrieve from the result set. Here’s an explanation with an example:
Consider a table named “employees” with columns: id, first_name, last_name, and age. Here’s a sample data:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 1 | John | Smith | 28 |
| 2 | Jane | Doe | 32 |
| 3 | Alex | Johnson | 24 |
| 4 | Lisa | Brown | 30 |
+----+------------+-----------+-----+
To retrieve a limited number of rows from this table, you can use the LIMIT keyword in the SELECT statement, like this:
SELECT * FROM employees LIMIT 2;
Output:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 1 | John | Smith | 28 |
| 2 | Jane | Doe | 32 |
+----+------------+-----------+-----+
In this example, the SELECT statement with the LIMIT keyword retrieves only the first 2 rows from the “employees” table. It restricts the result set to a maximum of 2 rows.
The LIMIT keyword is often used in conjunction with the ORDER BY clause to retrieve a specific number of top records based on a specified sorting criteria. Here’s an example:
SELECT * FROM employees ORDER BY age DESC LIMIT 3;
Output:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 2 | Jane | Doe | 32 |
| 4 | Lisa | Brown | 30 |
| 1 | John | Smith | 28 |
+----+------------+-----------+-----+
In this example, the SELECT statement retrieves the top 3 records from the “employees” table, sorted in descending order of the “age” column.
Different database systems may have variations in the syntax for limiting rows, so it’s recommended to refer to the specific documentation or resources of your database system for accurate syntax details and examples.
SQL: LIMIT OFFSET keyword, Explain with Example
In SQL, the LIMIT and OFFSET keywords are used together to control the number of rows returned by a query and to specify the starting point of the result set. The LIMIT clause sets the maximum number of rows to be returned, while the OFFSET clause defines the starting point or the number of rows to skip before returning results. Here’s an explanation with an example:
Consider a table named “employees” with columns: id, first_name, last_name, and age. Here’s a sample data:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 1 | John | Smith | 28 |
| 2 | Jane | Doe | 32 |
| 3 | Alex | Johnson | 24 |
| 4 | Lisa | Brown | 30 |
| 5 | Sarah | Davis | 35 |
+----+------------+-----------+-----+
To retrieve a specific number of rows from this table with an offset, you can use the LIMIT and OFFSET keywords in the SELECT statement. Here’s an example:
SELECT * FROM employees LIMIT 3 OFFSET 1;
Output:
+----+------------+-----------+-----+
| id | first_name | last_name | age |
+----+------------+-----------+-----+
| 2 | Jane | Doe | 32 |
| 3 | Alex | Johnson | 24 |
| 4 | Lisa | Brown | 30 |
+----+------------+-----------+-----+
In this example, the SELECT statement retrieves a maximum of 3 rows from the “employees” table starting from an offset of 1. It skips the first row and returns the subsequent three rows.
The OFFSET value determines the number of rows to skip before starting to return the result set. The LIMIT value sets the maximum number of rows to be included in the result set.
By adjusting the values of LIMIT and OFFSET, you can control the pagination of query results or retrieve subsets of data from larger result sets.
It’s important to note that the specific syntax and usage of the LIMIT and OFFSET keywords may vary slightly depending on the database management system (DBMS) you are using.
Technical content writer with data scientist, artificial intelligence, programming language, database. He has a bachelor’s degree in IT and a certificate in digital marketing, Digital transformation web development android app development He has written for website like Boomi techie, tech mantra, information hub, Tech all